Risk assessment for coastal flooding#
Click to open this workflow on Binder.
Click to go to this workflow’s GitHub repository.
In this workflow we will visualize risks to built infrastructure presented by coastal flooding. The damages will be calculated using the methodology described in the risk workflow description section\(^{1}\). We will use pre-processed coastal flood maps from the Global Flood Maps dataset and combine these with land use maps, as well as information on economic vulnerability (damage curves) to quantify the order of the damages in economic terms.
This methodology to calculating flood risk can also be used with other regional flood map datasets. In fact, for many locations the Global Flood Map dataset will not be accurate enough because it does not include coastal flood defences (i.e. some areas may appear flooded, while in reality there are flood defences in place to prevent that). But for initial understanding of how coastal flood risk can be estimated the global flood maps can be used.
Note: Country-specific information is required to calculate the economic damages. As a minimum, GDP should be adjusted in the accompanying Excel sheet. A copy of the Excel sheet will be made as part of this workflow and stored in the dedicated folder for regional assessment LUISA_damage_info_curves_[area name].xlsx.
\(^{1}\)see Risk_workflow_description_FLOOD_COASTAL.md
Preparation work#
Select area of interest#
Before accessing the data we will define the area of interest. Before starting with this workflow, you have already prepared by downloading the coastal flood hazard map to your local directory (using the hazard assessment workflow for coastal flooding or using your own data). Please specify below the area name for the coastal flood maps.
areaname = 'La_Rochelle'
Load libraries#
Find out about the Python libraries we will use in this notebook.
In this notebook we will use the following Python libraries:
os - Provides a way to interact with the operating system, allowing the creation of directories and file manipulation.
shutil - package for file operation (e.g. copying of files).
pooch - A data retrieval and storage utility that simplifies downloading and managing datasets.
numpy - A powerful library for numerical computations in Python, widely used for array operations and mathematical functions.
pandas - A data manipulation and analysis library, essential for working with structured data in tabular form.
rasterio - A library for reading and writing geospatial raster data, providing functionalities to explore and manipulate raster datasets.
rioxarray - An extension of the xarray library that simplifies working with geospatial raster data in GeoTIFF format.
damagescanner - A library designed for calculating flood damages based on geospatial data, particularly suited for analyzing flood impact.
matplotlib - A versatile plotting library in Python, commonly used for creating static, animated, and interactive visualizations.
contextily A library for adding basemaps to plots, enhancing geospatial visualizations.
cartopy A library for geospatial data processing.
shapely A library for manipulation and analysis of geometric objects.
These libraries collectively enable the download, processing, analysis, and visualization of geospatial and numerical data.
# Packages for downloading data and managing files
import os
import shutil
import pooch
# Packages for working with numerical data and tables
import numpy as np
import pandas as pd
# Packages for handling geospatial maps and data
import rioxarray as rxr
from rioxarray.merge import merge_datasets
import xarray as xr
import rasterio
from rasterio.enums import Resampling
# Package for calculating flood damages
from damagescanner.core import RasterScanner
# Ppackages used for plotting maps
import matplotlib.pyplot as plt
import contextily as ctx
import shapely.geometry
import cartopy.feature as cfeature
import cartopy.crs as ccrs
from pyproj import Transformer
Create the directory structure#
In order for this workflow to work even if you download and use just this notebook, we need to have the directory structure for accessing and storing data. If you have already executed the hazard assessment workflow for coastal flooding, you would already have created the workflow folder ‘FLOOD_COASTAL_hazard’ where the hazard data is stored. We create an additional folder for the risk workflow, called ‘FLOOD_COASTAL_risk’.
# Define folder containing hazard data
hazard_folder = 'FLOOD_COASTAL_hazard'
hazard_data_dir = os.path.join(hazard_folder, f'data_{areaname}')
# Define the folder for the risk workflow
workflow_folder = 'FLOOD_COASTAL_risk'
# Check if the workflow folder exists, if not, create it
if not os.path.exists(workflow_folder):
os.makedirs(workflow_folder)
# Define directories for data and results within the previously defined workflow folder
data_general_dir = os.path.join(workflow_folder,f'data_GENERAL')
data_dir = os.path.join(workflow_folder,f'data_{areaname}')
results_dir = os.path.join(workflow_folder, f'results_{areaname}')
plot_dir = os.path.join(workflow_folder, f'plot_{areaname}')
if not os.path.exists(data_general_dir):
os.makedirs(data_general_dir)
if not os.path.exists(data_dir):
os.makedirs(data_dir)
if not os.path.exists(results_dir):
os.makedirs(results_dir)
if not os.path.exists(plot_dir):
os.makedirs(plot_dir)
Download and explore the data#
Hazard data - coastal flood maps#
As default option, we use the potential coastal flood depth maps from the Global Flood Maps dataset, that were downloaded using the hazard assessment workflow for coastal floods.
Below we load the floodmaps and visualize them to check the contents.
floodmaps_path = os.path.join(hazard_data_dir,f'floodmaps_all_{areaname}.nc')
floodmaps = xr.open_dataset(floodmaps_path)
floodmaps.rio.write_crs("epsg:3035", inplace=True)
floodmaps
<xarray.Dataset> Dimensions: (x: 617, y: 643, year: 2, return_period: 6) Coordinates: * x (x) float64 3.425e+06 3.426e+06 ... 3.474e+06 3.474e+06 * y (y) float64 2.657e+06 2.657e+06 ... 2.607e+06 2.607e+06 * year (year) int32 2018 2050 * return_period (return_period) int32 2 5 10 50 100 250 spatial_ref int32 0 Data variables: inun (return_period, year, y, x) float32 ... Attributes: Conventions: CF-1.6 institution: Deltares project: Microsoft Planetary Computer - Global Flood Maps references: https://www.deltares.nl/en/ source: Global Tide and Surge Model v3.0 - ERA5
# make a plot of the first map in the dataset
fig, ax = plt.subplots(figsize=(7, 7))
floodmaps.isel(year=0,return_period=0)['inun'].plot(ax=ax,cmap='Blues',vmax=5)
ctx.add_basemap(ax=ax,crs=floodmaps.rio.crs,source=ctx.providers.CartoDB.Positron, attribution_size=6)
ax.set_title(f'{floodmaps.year.values[0]} \n 1 in {floodmaps.return_period.values[0]} years extreme event',fontsize=12)
Text(0.5, 1.0, '2018 \n 1 in 2 years extreme event')
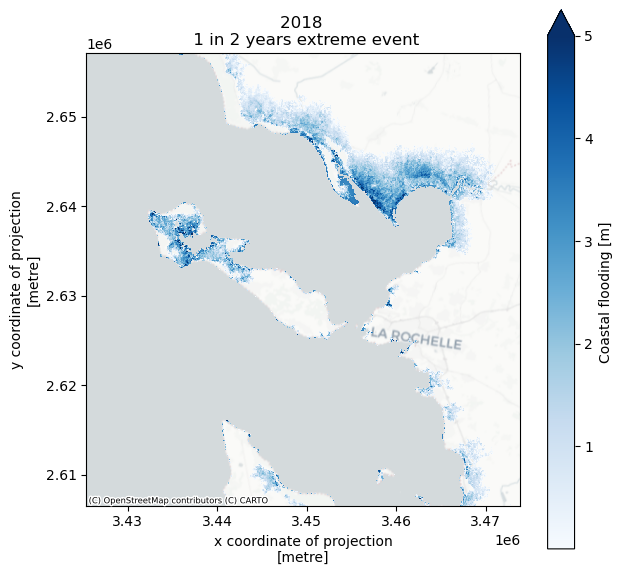
Based on the hazard map extent we will define the coordinates of the area of interest (in projected coordinates, EPSG:3035).
bbox = [np.min(floodmaps.x.values),np.min(floodmaps.y.values),np.max(floodmaps.x.values),np.max(floodmaps.y.values)]
bbox
[3425440.9274107153,
2606598.6145530045,
3473842.2071895604,
2657042.8054913464]
# convert to global spherical coordinates (WGS84)
transformer = Transformer.from_crs(floodmaps.rio.crs, "EPSG:4326")
bbox_global = list(transformer.transform(bbox[0],bbox[1])) + list(transformer.transform(bbox[2],bbox[3]))
bbox_global
[51.24470979029895,
-15.122934541142772,
51.80303346766081,
-14.666004092793864]
Exposure - land-use data#
Next we need the information on land use. We will download the land use dataset from the JRC data portal, a copy of the dataset will be saved locally for ease of access. In this notebook we use the land use maps with 100 m resolution. The land use maps can also be downloaded manually from the JRC portal.
landuse_res = 100 # choose resolution (options: 50 or 100 m)
luisa_filename = f'LUISA_basemap_020321_{landuse_res}m.tif'
# Check if land use dataset has not yet been downloaded
if not os.path.isfile(os.path.join(data_general_dir,luisa_filename)):
# , define the URL for the LUISA basemap and download it
url = f'http://jeodpp.jrc.ec.europa.eu/ftp/jrc-opendata/LUISA/EUROPE/Basemaps/2018/VER2021-03-24/{luisa_filename}'
pooch.retrieve(
url=url,
known_hash=None, # Hash value is not provided
path=data_general_dir, # Save the file to the specified data directory
fname=luisa_filename # Save the file with a specific name
)
else:
print(f'Land use dataset already downloaded at {data_general_dir}/{luisa_filename}')
Land use dataset already downloaded at FLOOD_COASTAL_risk\data_GENERAL/LUISA_basemap_020321_100m.tif
The Land use data is saved into the local data directory. The data shows on a 100 by 100 meter resolution what the land use is for Europe in 2018. The land use encompasses various types of urban areas, natural land, agricultural fields, infrastructure and waterbodies. This will be used as the first exposure layer in the risk assessment.
# Define the filename for the land use map based on the specified data directory
filename_land_use = f'{data_general_dir}/{luisa_filename}'
# Open the land use map raster
land_use = rxr.open_rasterio(filename_land_use)
# Display the opened land use map
land_use
<xarray.DataArray (band: 1, y: 46000, x: 65000)> [2990000000 values with dtype=int32] Coordinates: * band (band) int32 1 * x (x) float64 9e+05 9.002e+05 9.002e+05 ... 7.4e+06 7.4e+06 * y (y) float64 5.5e+06 5.5e+06 5.5e+06 ... 9.002e+05 9e+05 spatial_ref int32 0 Attributes: AREA_OR_POINT: Area _FillValue: 0 scale_factor: 1.0 add_offset: 0.0
The land use dataset needs to be clipped to the area of interest. For visualization purposes, each land use type is then assigned a color. Land use plot shows us the variation in land use over the area of interest.
# Set the coordinate reference system (CRS) for the land use map to EPSG:3035
land_use.rio.write_crs(3035, inplace=True)
# Clip the land use map to the specified bounding box and CRS
land_use_local = land_use.rio.clip_box(*bbox, crs=floodmaps.rio.crs)
# File to store the local land use map
landuse_map = os.path.join(data_dir, f'land_use_{areaname}.tif')
# Save the clipped land use map
with rasterio.open(
landuse_map,
'w',
driver='GTiff',
height=land_use_local.shape[1],
width=land_use_local.shape[2],
count=1,
dtype=str(land_use_local.dtype),
crs=land_use_local.rio.crs,
transform=land_use_local.rio.transform()
) as dst:
# Write the data array values to the rasterio dataset
dst.write(land_use_local.values)
# Plotting
# Define values and colors for different land use classes
LUISA_values = [1111, 1121, 1122, 1123, 1130,
1210, 1221, 1222, 1230, 1241,
1242, 1310, 1320, 1330, 1410,
1421, 1422, 2110, 2120, 2130,
2210, 2220, 2230, 2310, 2410,
2420, 2430, 2440, 3110, 3120,
3130, 3210, 3220, 3230, 3240,
3310, 3320, 3330, 3340, 3350,
4000, 5110, 5120, 5210, 5220,
5230]
LUISA_colors = ["#8c0000", "#dc0000", "#ff6969", "#ffa0a0", "#14451a",
"#cc10dc", "#646464", "#464646", "#9c9c9c", "#828282",
"#4e4e4e", "#895a44", "#a64d00", "#cd8966", "#55ff00",
"#aaff00", "#ccb4b4", "#ffffa8", "#ffff00", "#e6e600",
"#e68000", "#f2a64d", "#e6a600", "#e6e64d", "#c3cd73",
"#ffe64d", "#e6cc4d", "#f2cca6", "#38a800", "#267300",
"#388a00", "#d3ffbe", "#cdf57a", "#a5f57a", "#89cd66",
"#e6e6e6", "#cccccc", "#ccffcc", "#000000", "#ffffff",
"#7a7aff", "#00b4f0", "#50dcf0", "#00ffa6", "#a6ffe6",
"#e6f2ff"]
# Plot the land use map using custom levels and colors
land_use_local.plot(levels=LUISA_values, colors=LUISA_colors, figsize=(10, 8))
# Set the title for the plot
plt.title('LUISA Land Cover for the defined area');
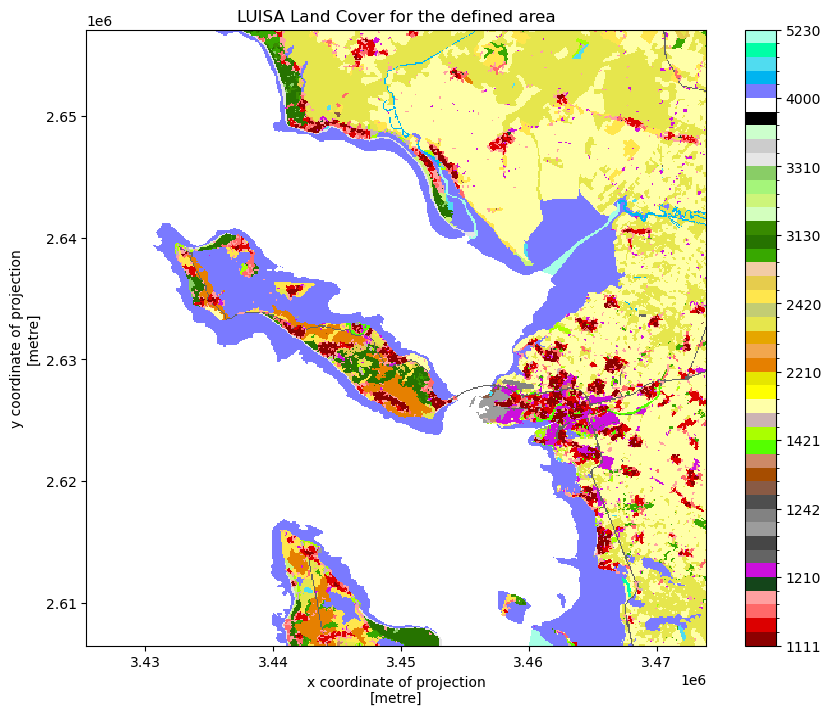
Vulnerability - damage curves for land use#
We will use damage curve files from the JRC that are already available in the GitHub repository folder.
# Import damage curves of the JRC from a CSV file into a pandas DataFrame
JRC_curves = pd.read_csv(os.path.join('../00_Input_files_for_damage_analysis','JRC_damage_curves.csv'), index_col=0)
# Plot the JRC depth-damage curves
JRC_curves.plot()
# Set the title and labels for the plot
plt.title('JRC depth-damage curves for different damage classes');
plt.xlabel('Flood depth [m]');
plt.ylabel('Damage ratio [%]');
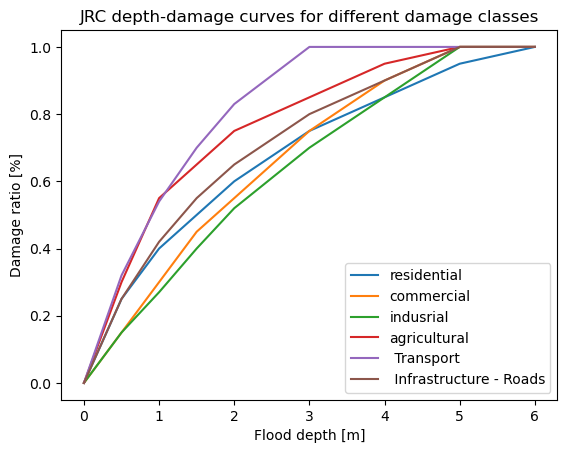
Processing data#
The maps of flooding and land use can be combined to assess multipe types of risk from coastal flooding in a region. This way we can estimate the exposure of infrastructure and economic assets to coastal floods. In this section we will align the resolutions of the datasets, prepare vulnerability curvers and calculate the damage maps.
Combining datasets with different resolution#
Before we can calculate risk indices, we will prepare the data by aligning the spatial resolution of the datasets and by calculating the vulnerability curves for economic damages based on specified information.
The flood and land use datasets have different spatial resolutions. Flood extent maps are at a resolution of 30-75 m (resolution varies with latitude), while land use data is at a constant 100 m (or 50 m) resolution. We can bring them to the same resolution. It is preferable to interpolate the flood map onto the land use grid (and not the other way around), because land use is defined in terms of discrete values and on a more convenient regularly spaced grid. We will interpolate the flood data onto the land use map grid in order to be able to calculate the damages.
# Reproject the flood map to match the resolution and extent of the land use map
years = floodmaps.year.values.tolist() # available scenario years
rps = floodmaps.return_period.values.tolist() # available return periods
for year in years:
for rp in rps:
ori_map = floodmaps['inun'].sel(year=year,return_period=rp)
new_map = ori_map.rio.reproject_match(land_use_local, resampling=Resampling.bilinear); del ori_map
ds = new_map.to_dataset(); del new_map
ds = ds.expand_dims(dim={'year':1,'return_period':1})
if (year==years[0]) & (rp==rps[0]):
floodmaps_resampled = ds
else:
floodmaps_resampled = floodmaps_resampled.merge(ds)
# check the new resolution of the floodmap (should be equivalent to the land use map resolution)
floodmaps_resampled.rio.resolution()
(100.0, -100.0)
We will save the resampled flood maps as raster files locally, so that we can more easily use them as input to calculate economic damages.
# Create GeoTIFF files for the resampled flood maps
tif_dir = os.path.join(data_dir,f'floodmaps_resampled')
if not os.path.isdir(tif_dir): os.makedirs(tif_dir)
for year in years:
for rp in rps:
data_tif = floodmaps_resampled['inun'].sel(year=year,return_period=rp,drop=True)
with rasterio.open(
f'{tif_dir}/floodmap_resampled_{areaname}_{year}_rp{rp:04d}.tif',
'w',
driver='GTiff',
height=data_tif.shape[0],
width=data_tif.shape[1],
count=1,
dtype=str(data_tif.dtype),
crs=data_tif.rio.crs,
transform=data_tif.rio.transform(),
) as dst:
# Write the data array values to the rasterio dataset
dst.write(data_tif.values,indexes=1)
Linking land use types to economic damages#
In order to assess the potential damage done by the flooding in a given scenario, we also need to assign a monetary value to the land use categories. We define this as the potential loss in €/m². The calculation of economic value for different land use types is made with help of an accompanying template (LUISA_damage_info_curves.xlsx).
damage_info_file = f'LUISA_damage_info_curves_{areaname}.xlsx'
template_file = os.path.join('..','00_Input_files_for_damage_analysis','LUISA_damage_info_curves_template.xlsx')
shutil.copyfile(template_file,os.path.join(data_dir,damage_info_file))
'FLOOD_COASTAL_risk\\data_La_Rochelle\\LUISA_damage_info_curves_La_Rochelle.xlsx'
Important: Please go to the newly created file and adjust the information on the GDP per capita in the first line of the Excel sheet.
# Read damage curve information from an Excel file into a pandas DataFrame
LUISA_info_damage_curve = pd.read_excel(os.path.join(data_dir,damage_info_file), index_col=0)
# Extract the 'total €/m²' column to get the maximum damage for reconstruction
maxdam = pd.DataFrame(LUISA_info_damage_curve['total €/m²'])
# Save the maximum damage values to a CSV file
maxdam_path = os.path.join(data_dir, f'maxdam_luisa.csv')
maxdam.to_csv(maxdam_path)
# Display the first 10 rows of the resulting DataFrame to view the result
maxdam.head(10)
total €/m² | |
---|---|
Land use code | |
1111 | 600.269784 |
1121 | 414.499401 |
1122 | 245.022999 |
1123 | 69.919184 |
1130 | 0.000000 |
1210 | 405.238393 |
1221 | 40.417363 |
1222 | 565.843080 |
1230 | 242.504177 |
1241 | 565.843080 |
# Create a new DataFrame for damage_curves_luisa by copying JRC_curves
damage_curves_luisa = JRC_curves.copy()
# Drop all columns in the new DataFrame
damage_curves_luisa.drop(damage_curves_luisa.columns, axis=1, inplace=True)
# Define building types for consideration
building_types = ['residential', 'commercial', 'industrial']
# For each land use class in maxdamage, create a new damage curve
for landuse in maxdam.index:
# Find the ratio of building types in the class
ratio = LUISA_info_damage_curve.loc[landuse, building_types].values
# Create a new curve based on the ratios and JRC_curves
damage_curves_luisa[landuse] = ratio[0] * JRC_curves.iloc[:, 0] + \
ratio[1] * JRC_curves.iloc[:, 1] + \
ratio[2] * JRC_curves.iloc[:, 2]
# Save the resulting damage curves to a CSV file
curve_path = os.path.join(data_dir, 'curves.csv')
damage_curves_luisa.to_csv(curve_path)
# Plot the vulnerability curves for the first 10 land cover types
damage_curves_luisa.iloc[:, 0:10].plot()
plt.title('Vulnerability curves for flood damages for the LUISA land cover types');
plt.ylabel('Damage (%)');
plt.xlabel('Inundation depth (m)');
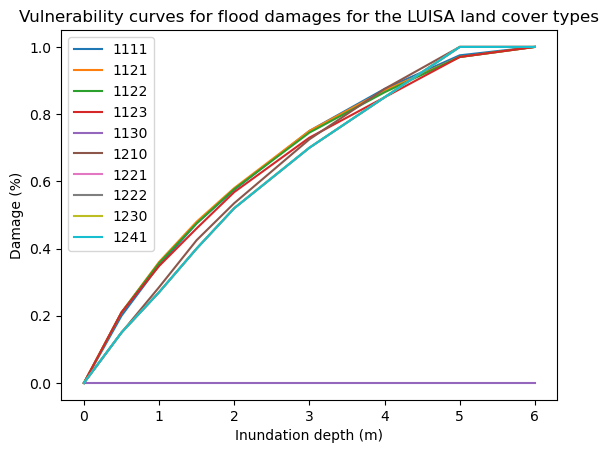
Calculate potential economic damage to infrastructure using DamageScanner#
Now that we have all pieces of the puzzle in place, we can perform the risk calculation. For this we are using the DamageScanner python library which allows for an easy damage calculation.
The DamageScanner takes the following data:
The clipped and resampled flood map
The clipped land use map
The vulnerability curves per land use category
A table of maximum damages per land use category
We can perform the damage calculations for all scenarios and return periods now:
for year in years:
for rp in rps:
inun_map = os.path.join(tif_dir, f'floodmap_resampled_{areaname}_{year}_rp{rp:04}.tif') # Define file path for the flood map input
# Do the damage calculation and save the results
loss_df = RasterScanner(landuse_map,
inun_map,
curve_path,
maxdam_path,
save = True,
nan_value = None,
scenario_name= '{}/flood_scenario_{}_{}_rp{:04}'.format(results_dir,areaname,year,rp),
dtype = np.int64)
loss_df_renamed = loss_df[0].rename(columns={"damages": "{}_rp{:04}".format(year,rp)})
if (year==years[0]) & (rp==rps[0]):
loss_df_all = loss_df_renamed
else:
loss_df_all = pd.concat([loss_df_all, loss_df_renamed], axis=1)
Now the dataframe loss_df_all contains the results of damage calculations for all scenarios and return periods. We will format this dataframe for easier interpretation:
# Obtain the LUISA legend and add it to the table of damages
LUISA_legend = LUISA_info_damage_curve['Description']
# Convert the damages to million euros
loss_df_all_mln = loss_df_all / 10**6
# Combine loss_df with LUISA_legend
category_damage = pd.concat([LUISA_legend, (loss_df_all_mln)], axis=1)
# Sort the values by damage in descending order (based on the column with the highest damages)
category_damage.sort_values(by=category_damage.columns[-1], ascending=False, inplace=True)
category_damage=category_damage.round(1)
# Display the resulting DataFrame (top 10 rows)
category_damage.head(10)
Description | 2018_rp0002 | 2018_rp0005 | 2018_rp0010 | 2018_rp0050 | 2018_rp0100 | 2018_rp0250 | 2050_rp0002 | 2050_rp0005 | 2050_rp0010 | 2050_rp0050 | 2050_rp0100 | 2050_rp0250 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
2110 | Non irrigated arable land | 2034.0 | 2325.3 | 2562.3 | 3148.7 | 3422.9 | 3809.1 | 2271.3 | 2581.8 | 2826.5 | 3443.6 | 3735.4 | 4133.2 |
2310 | Pastures | 663.4 | 849.5 | 986.4 | 1322.8 | 1524.8 | 1736.8 | 817.9 | 996.1 | 1137.7 | 1534.7 | 1695.4 | 1907.6 |
4000 | Wetlands | 1338.0 | 1435.2 | 1510.5 | 1670.5 | 1741.2 | 1827.8 | 1416.7 | 1515.1 | 1583.0 | 1745.2 | 1812.1 | 1889.8 |
1111 | High density urban fabric | 279.4 | 402.2 | 490.0 | 757.8 | 899.4 | 1119.8 | 385.2 | 497.9 | 598.7 | 908.9 | 1079.1 | 1286.3 |
1121 | Medium density urban fabric | 336.4 | 417.0 | 481.9 | 647.5 | 733.9 | 851.4 | 400.3 | 485.8 | 558.9 | 738.6 | 826.5 | 951.7 |
1210 | Industrial or commercial units | 239.8 | 288.3 | 325.1 | 418.2 | 462.3 | 521.0 | 281.5 | 331.2 | 369.0 | 468.4 | 513.2 | 575.0 |
1122 | Low density urban fabric | 198.5 | 243.7 | 276.3 | 361.6 | 400.4 | 463.9 | 235.9 | 277.9 | 315.4 | 403.2 | 454.5 | 510.1 |
2420 | Complex cultivation patterns | 229.7 | 258.5 | 279.9 | 332.6 | 371.3 | 405.7 | 253.0 | 281.1 | 302.4 | 373.0 | 399.3 | 432.6 |
1123 | Isolated or very low density urban fabric | 139.4 | 164.8 | 184.5 | 237.0 | 264.0 | 299.3 | 159.8 | 185.7 | 208.1 | 265.2 | 292.1 | 324.5 |
1422 | Sport and leisure built-up | 129.7 | 152.9 | 168.0 | 207.8 | 236.0 | 263.1 | 150.0 | 170.1 | 187.8 | 237.9 | 260.8 | 281.4 |
Plot the results#
Now we can plot the damages to get a spatial view of what places can potentially be most affected economically. To do this, first the damage maps for all scenarios will be loaded into memory and formatted:
# load all damage maps and merge into one dataset
for year in years:
for rp in rps:
damagemap = rxr.open_rasterio('{}/flood_scenario_{}_{}_rp{:04}_damagemap.tif'.format(results_dir,areaname,year,rp)).squeeze()
damagemap = damagemap.where(damagemap > 0)/10**6
damagemap.load()
# prepare for merging
damagemap.name = 'damages'
damagemap = damagemap.assign_coords(year=year);
damagemap = damagemap.assign_coords(return_period=rp);
ds = damagemap.to_dataset(); del damagemap
ds = ds.expand_dims(dim={'year':1,'return_period':1})
# merge
if (year==years[0]) & (rp==rps[0]):
damagemap_all = ds
else:
damagemap_all = damagemap_all.merge(ds)
damagemap_all.x.attrs['long_name'] = 'X coordinate'; damagemap_all.x.attrs['units'] = 'm'
damagemap_all.y.attrs['long_name'] = 'Y coordinate'; damagemap_all.y.attrs['units'] = 'm'
damagemap_all.load()
<xarray.Dataset> Dimensions: (x: 485, y: 506, year: 2, return_period: 6) Coordinates: * x (x) float64 3.425e+06 3.426e+06 ... 3.474e+06 3.474e+06 * y (y) float64 2.657e+06 2.657e+06 ... 2.607e+06 2.607e+06 * year (year) int32 2018 2050 * return_period (return_period) int32 2 5 10 50 100 250 band int32 1 spatial_ref int32 0 Data variables: damages (year, return_period, y, x) float64 nan nan nan ... nan nan
Now we can plot the damage maps to compare:
damagemap_all.isel(year=-1,return_period=-1)
<xarray.Dataset> Dimensions: (x: 485, y: 506) Coordinates: * x (x) float64 3.425e+06 3.426e+06 ... 3.474e+06 3.474e+06 * y (y) float64 2.657e+06 2.657e+06 ... 2.607e+06 2.607e+06 year int32 2050 return_period int32 250 band int32 1 spatial_ref int32 0 Data variables: damages (y, x) float64 nan nan nan nan nan ... nan nan nan nan nan
# select return periods to plot
rps_sel = [2,10,100]
# Plot damage maps for different scenarios and return periods
fig,axs = plt.subplots(figsize=(10, 10/len(rps_sel)*2),nrows=len(years),ncols=len(rps_sel),constrained_layout=True,sharex=True,sharey=True)
# define limits for the damage axis based on the map with highest damages
vrange = [0,np.nanmax(damagemap_all.isel(year=-1,return_period=-1)['damages'].values)]
#subfigs = fig.subfigures(len(years), 1)
for yy,year in enumerate(years):
for rr,rp in enumerate(rps_sel):
# Plot the damagemap with a color map representing damages and a color bar
bs=damagemap_all.sel(year=year,return_period=rp)['damages'].plot(ax=axs[yy,rr], vmin=vrange[0], vmax=vrange[1], cmap='Reds', add_colorbar=False)
ctx.add_basemap(axs[yy,rr],crs=damagemap_all.rio.crs.to_string(),source=ctx.providers.CartoDB.Positron, attribution_size=6) # add basemap
axs[yy,rr].set_title(f'{year} \n 1 in {rp} years extreme event',fontsize=12)
if rr>0:
axs[yy,rr].yaxis.label.set_visible(False)
if yy==0:
axs[yy,rr].xaxis.label.set_visible(False)
fig.colorbar(bs,ax=axs[:],orientation="vertical",pad=0.01,shrink=0.9,aspect=30).set_label(label=f'Damage [mln. €]',size=14)
fig.suptitle('Coastal flood damages for extreme sea water level scenarios',fontsize=12);
fileout = os.path.join(plot_dir,'Result_map_{}_damages_overview.png'.format(areaname))
fig.savefig(fileout)
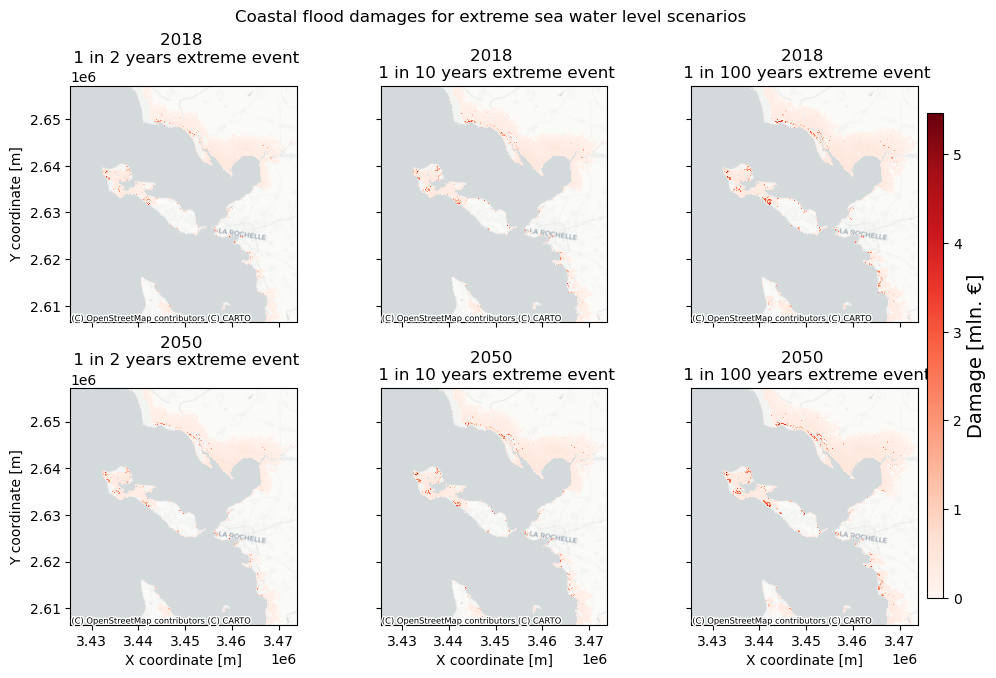
To get a better indication of why certain areas are damaged more than others, we can also plot the floodmap and land use maps in one figure for a given return period.
# Select year and return period to plot:
year = 2050
rp = 100
# load damage map
damagemap = rxr.open_rasterio('{}/flood_scenario_{}_{}_rp{:04}_damagemap.tif'.format(results_dir,areaname,year,rp))
damagemap = damagemap.where(damagemap > 0)/10**6
fig, ([ax1, ax2, ax3]) = plt.subplots(figsize=(13, 4),nrows=1,ncols=3,sharex=True,sharey=True,layout='constrained')
# Plot flood damages on the first plot
damagemap.plot(ax=ax1, cmap='Reds', cbar_kwargs={'label': "Damage [mln. €]",'pad':0.01,'shrink':0.95,'aspect':30})
ax1.set_title(f'Flood damages for 1 in {rp} year return period')
ax1.set_xlabel('X coordinate in the projection'); ax1.set_ylabel('Y coordinate in the projection')
# Plot inundation depth on the second plot
floodmaps_resampled.sel(year=year,return_period=rp)['inun'].plot(ax=ax2, cmap='Blues', cbar_kwargs={'label': "Inundation depth [m]",'pad':0.01,'shrink':0.95,'aspect':30})
ax2.set_title(f'Flood depth for 1 in {rp} years extreme event')
ax2.set_xlabel('X coordinate in the projection'); ax2.set_ylabel('Y coordinate in the projection')
ax2.yaxis.label.set_visible(False)
# Plot land use on the third plot with custom colors
land_use_local.plot(ax=ax3, levels=LUISA_values, colors=LUISA_colors, cbar_kwargs={'label': "Land use class [-]",'pad':0.01,'shrink':0.95,'aspect':30})
ax3.set_title('LUISA Land Cover for the defined area')
ax3.set_xlabel('X coordinate in the projection'); ax3.set_ylabel('Y coordinate in the projection')
ax3.yaxis.label.set_visible(False)
plt.suptitle('Flood maps for extreme sea water level scenarios in ' + f'year {year}',fontsize=12)
# Add a map background to each plot using Contextily
ctx.add_basemap(ax1, crs=damagemap.rio.crs.to_string(), source=ctx.providers.CartoDB.Positron)
ctx.add_basemap(ax2, crs=floodmaps_resampled.rio.crs.to_string(), source=ctx.providers.CartoDB.Positron)
ctx.add_basemap(ax3, crs=land_use_local.rio.crs.to_string(), source=ctx.providers.CartoDB.Positron)
# Display the plot
plt.show()
fileout = os.path.join(plot_dir,'Result_map_{}_{}_rp{:04}.png'.format(areaname,year,rp))
fig.savefig(fileout)
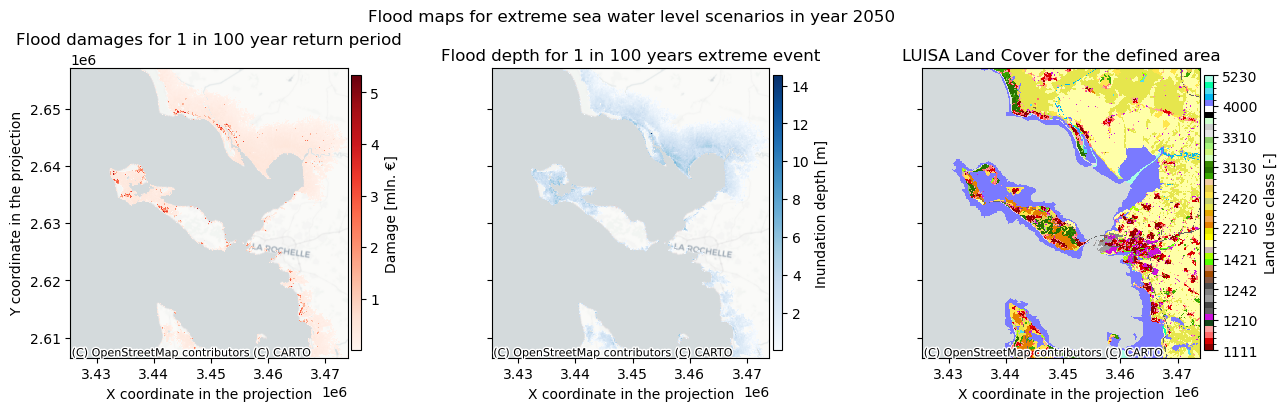
Here we see both the the potential flood depths and the associated economic damages. This overview helps to see which areas carry the most economic risk under the flooding scenarios.
Make sure to check the results and try to explain why high damages do or do not occur in case of high innundation. Find that something is wrong? Reiterate your assumptions made in the LUISA_damage_info_curves.xlsx and run the workflow again.
Conclusions#
Now that you were able to calculate damage maps based on flood maps and view the results, it is time to revisit the information about the accuracy and applicability of global flood maps to local contexts (see section Global Flood Maps dataset and its applicability for local risk assessment in the risk workflow description).
Consider the following questions:
How accurate do you think this result is for your local context? Are there geographical and/or infrastructural factors that make this result less accurate?
What information are you missing that could make this assessment more accurate?
What can you already learn from these maps of coastal flood potential and maps of potential damages?
Important
In this risk workflow we learned:
How to access use European-scale land use datasets.
How to assign each land use with a vulnerability curve and maximum damage.
Combining the flood (hazard), land use (exposure), and the vulnerability curves (vulnerability) to obtain an economic damage estimate.
Understand where damage comes from and how exposure and vulnerability are an important determinant of risk.
Contributors#
Applied research institute Deltares (The Netherlands).
Authors of the workflow:
Natalia Aleksandrova
Ted Buskop
Global Flood Maps dataset documentation can be found here.